ndarray에 대한 설명은 이전 포스팅을 참고하면 된다.
2024.09.09 - [공부한 것들../Numpy] - Numpy 자료구조 :: ndarray (N- Dimension Array)
Numpy 자료구조 :: ndarray (N- Dimension Array)
# Numpy 자료구조 :: ndarrayNumpy 라이브러리의 주요 자료구조는 ndarray로 다차원 데이터를 저장하며 여러 연산 및 인덱싱에 필요한 툴을 제공한다.위의 사진은 n차원의 배열을 시각화하여 표현한 것
bottle-an00.tistory.com
# ndarray 생성 방법
ndarray 생성 방법은 아래와 같은 방법이 있다. 여러 방법에 대한 api reference를 각각 살펴보고 예시 코드를 실행해보아 ndarray 생성 방법을 이해하고 정리한다.
- np.array
- np.arange
- np.zero
- np.ones
- np.full
- np.empty
- np.*_like
# API Reference 살펴보기
# numpy.array
API Reference:: numpy 홈페이지 API Reference :: numpy.array
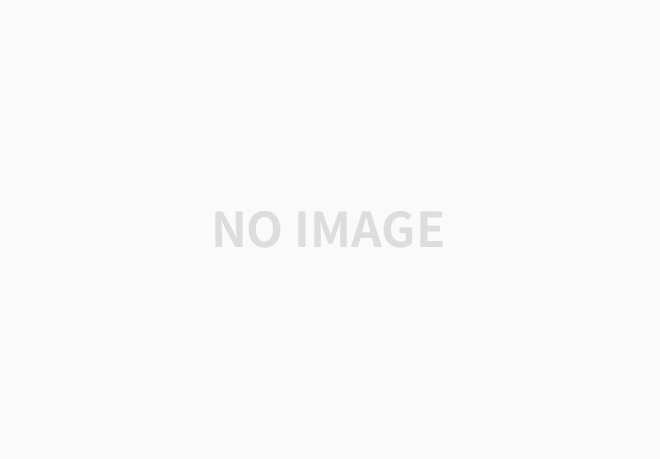
※ numpy.array() 파라미터
- object : array_like:배열을 생성할 때 사용하는 입력 데이터. 리스트, 튜플 등 배열로 변환 가능한 객체를 입력할 수 있다
- dtype: 배열 요소의 데이터 타입을 지정한다. 따로 값을 지정하지 않으면 자동으로 적절한 타입을 선택한다.
- copy: True일 경우 배열 데이터를 복사한다. None일 경우, 다른 조건에 따라 복사가 필요할 때만 복사한다.
- order: 배열의 메모리 레이아웃을 지정한다.
- 'K': 기존 배열의 순서를 유지.
- 'A': 입력이 Fortran 순서면 Fortran 순서, 아니면 C 순서.
- 'C': C 순서 (행 우선).'F': Fortran 순서 (열 우선).
- subok: True일 경우, 하위 클래스도 유지된다. False일 경우, 기본 NumPy 배열로 변환된다.
- ndmin: 결과 배열의 최소 차원 수를 지정한다. 입력 객체의 차원이 부족할 경우, 1로 채워서 차원을 맞춘다.
- like: NumPy 1.20부터 추가된 기능으로, 배열과 호환되는 객체를 기준으로 배열을 생성한다.
※ numpy.array() 예시 코드
# Example 01
import numpy as np
#1차원 데이터
data_1D = [1,2,3]
test_arr1 = np.array(data_1D)
print("\n1D array info:: ")
print("data::",test_arr1)
print("shape:: {}, size::{} dtype::{} dimension::{}"
.format(test_arr1.shape, test_arr1.size, test_arr1.dtype, test_arr1.ndim))
#2차원 데이터
data_2D = [[1,2,3],[3,4,4]]
test_arr2 = np.array(data_2D)
print("\n2D array info:: ")
print("data::",test_arr2)
print("shape:: {}, size::{} dtype::{} dimension::{}"
.format(test_arr2.shape, test_arr2.size, test_arr2.dtype, test_arr2.ndim))
#Example01 실행 결과
1D array info::
data:: [1 2 3]
shape:: (3,), size::3 dtype::int64 dimension::1
2D array info::
data:: [[1 2 3]
[3 4 4]]
shape:: (2, 3), size::6 dtype::int64 dimension::2
# Example 02 :: Upcasting 확인
#Upcasting 확인
test_arr3 = np.array([1, 2, 3.0])
print("\ntest_arr3 type:: ", test_arr3.dtype)
#Example02 결과
test_arr3 type:: float64
# Example 03:: ndim 파라미터 설정
#ndim 파라미터 설정
test_arr4 = np.array([1, 2, 3], ndmin=2)
print("\ntest_arr4 check minimum ndim:: ", test_arr4.ndim)
test_arr4 check minimum ndim:: 2
# Example 04:: type 파라미터 설정
#dtype 파라미터 설정
test_arr5 = np.array([1, 2, 3], dtype=complex)
print("\ntest_arr5 dtype:: ", test_arr5.dtype)
#Example04결과
test_arr5 dtype:: complex128
# Example 05 :: 다중 요소로 구성된 array data type설정
#다중 요소로 구성된 array data type설정
test_arr6 = np.array([(1,2),(3,4)],dtype=[('a','<i4'),('b','<i4')])
print("\nData-type consisting of more than one element:")
print("print test_arr6['a']",test_arr6['a'])
print("print test_arr6['b']",test_arr6['b'])
#Example05 결과
Data-type consisting of more than one element:
print test_arr6['a'] [1 3]
print test_arr6['b'] [2 4]
# Example 06 :: subok 파라미터 설정
#subok 파라미터 설정
test_arr7 = np.array(np.asmatrix('1 2; 3 4'))
print("\nCreating an array from sub-classes:")
print("subok = False :: ",type(test_arr7))
test_arr8 = np.array(np.asmatrix('1 2; 3 4'), subok=True)
print("subok = True :: ",type(test_arr8.dtype))
#Example06결과
Creating an array from sub-classes:
subok = False :: <class 'numpy.ndarray'>
subok = True :: <class 'numpy.dtype[int64]'>
# numpy.arange
API Reference:: numpy 홈페이지 API Reference :: numpy.arange
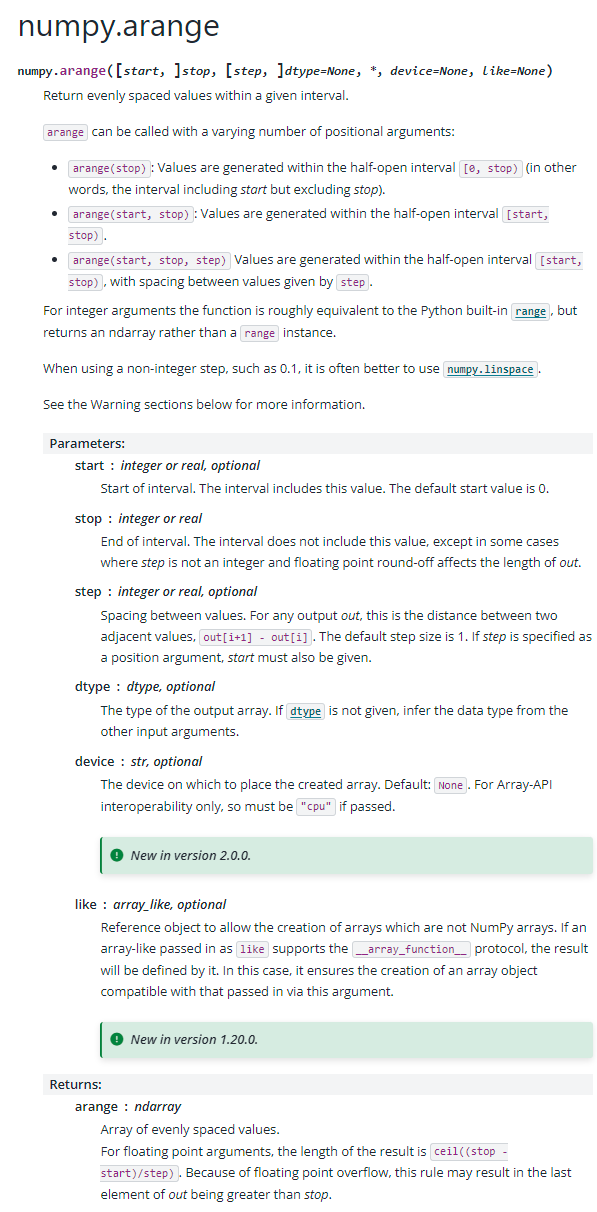
※ numpy.arange() 파라미터
- start: 구간의 시작 값으로, 이 값은 구간에 포함된다. 기본 시작 값은 0이다.
- stop: 구간의 끝 값으로, 이 값은 구간에 포함되지 않는다. 단, step이 정수가 아니거나 부동 소수점 반올림으로 인해 출력 길이에 영향을 미치는 경우에는 포함될 수 있다.
- step: 값 사이의 간격을 정의하며, 출력 배열에서 두 인접 값 사이의 거리(out[i+1] - out[i])를 의미한다. 기본 간격은 1이다. step이 위치 인자로 지정된 경우, start 값도 반드시 제공되어야 한다.
- dtype: 출력 배열의 데이터 타입을 지정한다. dtype이 지정되지 않은 경우, 다른 입력 인자에서 데이터 타입을 유추한다.
- device: 생성된 배열을 배치할 장치를 지정하며, 기본값은 None이다. Array-API 호환성만을 위해 사용되며, 전달 시 "cpu"로 설정해야 한다.
- like: NumPy 배열이 아닌 배열을 생성할 수 있도록 하는 참조 객체이다. like로 전달된 배열이 __array_function__ 프로토콜을 지원하면, 결과는 이 프로토콜에 의해 정의된다. 이 경우, 전달된 객체와 호환되는 배열 객체가 생성된다.
※ numpy.arange() 예시 코드
# Example
import numpy as np
def printinfo(arr):
print(arr)
print("shape:: {}, size::{} dtype::{} dimension::{}"
.format(arr.shape, arr.size, arr.dtype, arr.ndim))
test_array1 = np.arange(2,10)
print("Test array1:: set start, stop")
printinfo(test_array1)
test_array2 = np.arange(9.0)
print("Test array2:: set only stop parameter")
printinfo(test_array2)
test_array3 = np.arange(2, 10, 2)
print("Test array3:: set start, stop, step")
printinfo(test_array3)
Test array3:: set start, stop
[2 3 4 5 6 7 8 9]
shape:: (8,), size::8 dtype::int64 dimension::1
Test array2:: set only stop parameter
[0. 1. 2. 3. 4. 5. 6. 7. 8.]
shape:: (9,), size::9 dtype::float64 dimension::1
Test array3:: set start, stop, step
[2 4 6 8]
shape:: (4,), size::4 dtype::int64 dimension::1
# numpy.zeros
API Reference:: numpy 홈페이지 API Reference :: numpy.zeros
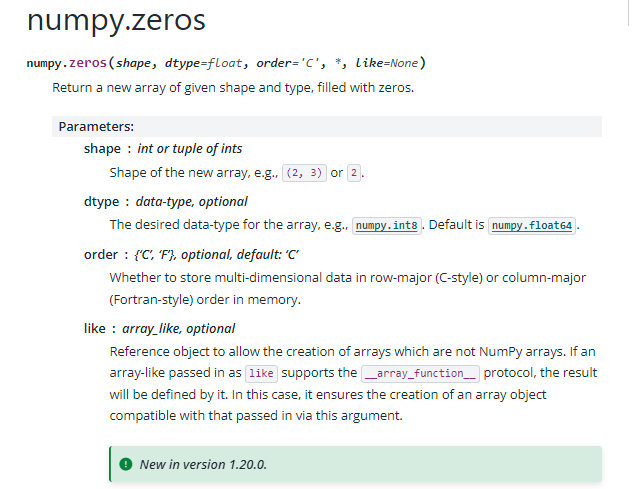
※ numpy.zeros() 파라미터
- shape : 새 배열의 형태를 정의하며, 예를 들어 (2, 3) 또는 2와 같이 지정할 수 있다.
- order : 다차원 데이터를 메모리에 저장하는 방식을 지정하며, ‘C’는 행 우선(C-style) 저장, ‘F’는 열 우선(Fortran-style) 저장을 의미한다. 기본값은 ‘C’이다.
- like : NumPy 배열이 아닌 배열을 생성할 수 있도록 하는 참조 객체이다. like로 전달된 배열이 __array_function__ 프로토콜을 지원하면, 결과는 이 프로토콜에 의해 정의된다. 이 경우, 전달된 객체와 호환되는 배열 객체가 생성된다.
- dtype : 배열의 원하는 데이터 타입을 지정한다. 예를 들어 numpy.int8을 사용할 수 있다. 기본값은 numpy.float64이다.
※ numpy.zeros() 예시 코드
# Example
import numpy as np
def printinfo(arr):
print(arr)
print("shape:: {}, size::{} dtype::{} dimension::{}"
.format(arr.shape, arr.size, arr.dtype, arr.ndim))
test_array1 = np.zeros(10)
print("Test array1:: set shape")
printinfo(test_array1)
test_array2 = np.zeros((2,4), dtype=int)
print("Test array2:: set shape and dtype")
printinfo(test_array2)
test_array3 = np.zeros((2,), dtype=[('x', 'i4'), ('y', 'i4')])
print("Test array3:: set shape, custom dtype")
printinfo(test_array3)
Test array1:: set shape
[0. 0. 0. 0. 0. 0. 0. 0. 0. 0.]
shape:: (10,), size::10 dtype::float64 dimension::1
Test array2:: set shape and dtype
[[0 0 0 0]
[0 0 0 0]]
shape:: (2, 4), size::8 dtype::int64 dimension::2
Test array3:: set shape, custom dtype
[(0, 0) (0, 0)]
shape:: (2,), size::2 dtype::[('x', '<i4'), ('y', '<i4')] dimension::1
# numpy.ones()
API Reference:: numpy 홈페이지 API Reference :: numpy.ones
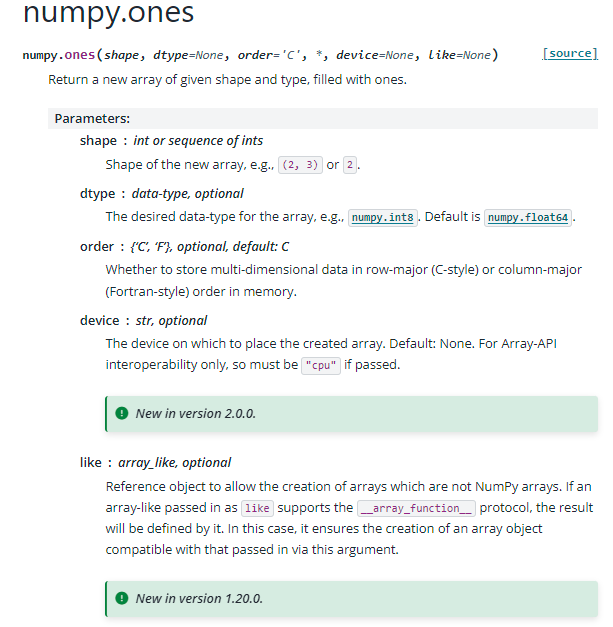
※ numpy.ones() 파라미터
- shape : 새 배열의 형태를 정의하며, 예를 들어 (2, 3) 또는 2와 같이 지정할 수 있다.
- order : 다차원 데이터를 메모리에 저장하는 방식을 지정하며, ‘C’는 행 우선(C-style) 저장, ‘F’는 열 우선(Fortran-style) 저장을 의미한다. 기본값은 ‘C’이다.
- like : NumPy 배열이 아닌 배열을 생성할 수 있도록 하는 참조 객체이다. like로 전달된 배열이 __array_function__ 프로토콜을 지원하면, 결과는 이 프로토콜에 의해 정의된다. 이 경우, 전달된 객체와 호환되는 배열 객체가 생성된다.
- dtype : 배열의 원하는 데이터 타입을 지정한다. 예를 들어 numpy.int8을 사용할 수 있다. 기본값은 numpy.float64이다.
※ numpy.ones() 예시 코드
# Example
import numpy as np
def printinfo(arr):
print(arr)
print("shape:: {}, size::{} dtype::{} dimension::{}"
.format(arr.shape, arr.size, arr.dtype, arr.ndim))
test_array1 = np.ones((3,4))
print("Test array1:: set shape")
printinfo(test_array1)
test_array2 = np.ones((2,4), dtype=int)
print("Test array2:: set shape and dtype")
printinfo(test_array2)
Test array1:: set shape
[[1. 1. 1. 1.]
[1. 1. 1. 1.]
[1. 1. 1. 1.]]
shape:: (3, 4), size::12 dtype::float64 dimension::2
Test array2:: set shape and dtype
[[1 1 1 1]
[1 1 1 1]]
shape:: (2, 4), size::8 dtype::int64 dimension::2
# numpy.full()
API Reference:: numpy 홈페이지 API Reference :: numpy.full

※ numpy.full() 파라미터
- shape : 새 배열의 형태를 정의하며, 예를 들어 (2, 3) 또는 2와 같이 지정할 수 있다.
- fill_value : 배열을 채울 값으로, 스칼라나 배열 같은 값을 사용할 수 있다.
- dtype : 배열의 원하는 데이터 타입을 지정한다. 기본값인 None은 np.array(fill_value).dtype를 의미한다.
- order : 다차원 데이터를 메모리에 저장하는 방식을 지정하며, ‘C’는 행 우선(C-style) 저장, ‘F’는 열 우선(Fortran-style) 저장을 의미한다.
- device : 생성된 배열을 배치할 장치를 지정하며, 기본값은 None이다. Array-API 호환성만을 위해 사용되며, 전달 시 "cpu"로 설정해야 한다.
- like : NumPy 배열이 아닌 배열을 생성할 수 있도록 하는 참조 객체이다. like로 전달된 배열이 __array_function__ 프로토콜을 지원하면, 결과는 이 프로토콜에 의해 정의된다. 이 경우, 전달된 객체와 호환되는 배열 객체가 생성된다.
※ numpy.full() 예시 코드
# Example
import numpy as np
def printinfo(arr):
print(arr)
print("shape:: {}, size::{} dtype::{} dimension::{}"
.format(arr.shape, arr.size, arr.dtype, arr.ndim))
test_array1 = np.full((4,4), np.inf)
print("Test array1:: set shape and fill_value1")
printinfo(test_array1)
test_array2 = np.full((2,2), [6,2])
print("Test array2:: set shape and fill_value2")
printinfo(test_array2)
Test array1:: set shape and fill_value1
[[inf inf inf inf]
[inf inf inf inf]
[inf inf inf inf]
[inf inf inf inf]]
shape:: (4, 4), size::16 dtype::float64 dimension::2
Test array2:: set shape and fill_value2
[[6 2]
[6 2]]
shape:: (2, 2), size::4 dtype::int64 dimension::2
# numpy.empty()
API Reference:: numpy 홈페이지 API Reference :: numpy.empty
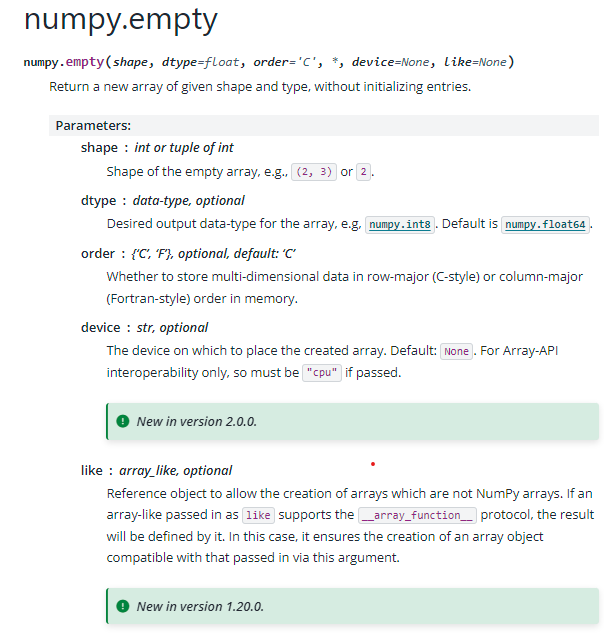
numpy.empty()는 요소들의 초기화 없이 배열을 생성하는 것으로 생성 비용이 가장 저렴하고 빠르다.
생성된 배열의 각 요소는 임의의 값으로 저장되어있다.
※ numpy.empty() 파라미터
- shape : 빈 배열의 형태를 정의하며, 예를 들어 (2, 3) 또는 2와 같이 지정할 수 있다.
- dtype : 배열의 원하는 데이터 타입을 지정한다. 예를 들어 numpy.int8을 사용할 수 있다. 기본값은 numpy.float64이다.
- order : 다차원 데이터를 메모리에 저장하는 방식을 지정하며, ‘C’는 행 우선(C-style) 저장, ‘F’는 열 우선(Fortran-style) 저장을 의미한다. 기본값은 ‘C’이다.
- device : 생성된 배열을 배치할 장치를 지정하며, 기본값은 None이다. Array-API 호환성만을 위해 사용되며, 전달 시 "cpu"로 설정해야 한다.
- like : NumPy 배열이 아닌 배열을 생성할 수 있도록 하는 참조 객체이다. like로 전달된 배열이 __array_function__ 프로토콜을 지원하면, 결과는 이 프로토콜에 의해 정의된다. 이 경우, 전달된 객체와 호환되는 배열 객체가 생성된다.
※ numpy.empty() 예시 코드
# Example
import numpy as np
def printinfo(arr):
print(arr)
print("shape:: {}, size::{} dtype::{} dimension::{}"
.format(arr.shape, arr.size, arr.dtype, arr.ndim))
test_array1 = np.empty((2,2))
print("Test array1:: set shape")
printinfo(test_array1)
test_array2 = np.empty((2,2), dtype=int)
print("Test array2:: set shape and dtype")
printinfo(test_array2)
Test array1:: set shape
[[4.65314289e-310 0.00000000e+000]
[2.76676762e-322 4.65311237e-310]]
shape:: (2, 2), size::4 dtype::float64 dimension::2
Test array2:: set shape and dtype
[[94180660668208 0]
[ 56 94180042866688]]
shape:: (2, 2), size::4 dtype::int64 dimension::2
# numpy.*_like()
numpy.*_like()종류는 다음과 같다.
- numpy.zeros_like()
- numpy.ones_like()
- numpy.full_like()
- numpy.empty_like()
numpy.*_like()는 지정된 배열과 shape이 같은 각 함수의 특징으로 ndarray를 생성한다는 공통점이 존재한다. 대표적으로 numpy.ones_like()를 살펴보자
API Reference:: numpy 홈페이지 API Reference :: numpy.ones_like
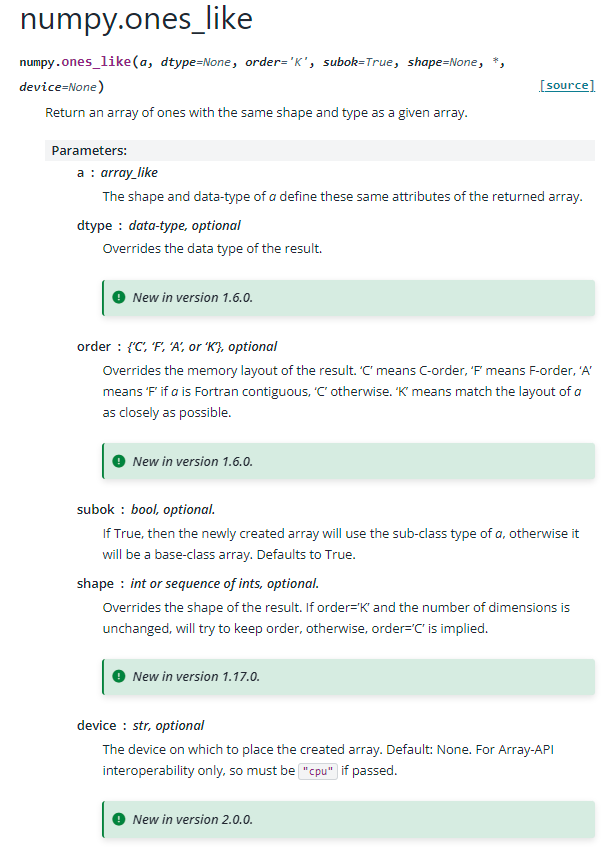
※ numpy.ones_like() 파라미터
- a : 배열과 유사한 객체로, a의 형태와 데이터 타입이 반환된 배열의 동일한 속성을 정의한다.
- order : 결과의 메모리 레이아웃을 재정의할 수 있다. ‘C’는 C-order를 의미하며, ‘F’는 F-order를 의미한다. ‘A’는 a가 Fortran 연속이면 ‘F’, 그렇지 않으면 ‘C’를 사용한다. ‘K’는 a의 레이아웃과 가능한 한 가깝게 맞추려고 한다.
- shape : 결과 배열의 형태를 재정의할 수 있다. order=’K’이고 차원이 변경되지 않는 경우, 레이아웃을 유지하려고 시도하며, 그렇지 않으면 order=’C’가 암시된다.
- device : 생성된 배열을 배치할 장치를 지정하며, 기본값은 None이다. Array-API 호환성만을 위해 사용되며, 전달 시 "cpu"로 설정해야 한다.
- subok : 새로 생성된 배열이 a의 서브클래스 타입을 사용할지 여부를 결정한다. True일 경우 서브클래스 타입을 사용하며, 기본값은 True이다. False일 경우 기본 클래스 배열이 된다.
- dtype : 결과 배열의 데이터 타입을 재정의할 수 있다.
※ numpy.ones_like() 예시 코드
# Example
import numpy as np
def printinfo(arr):
print(arr)
print("shape:: {}, size::{} dtype::{} dimension::{}"
.format(arr.shape, arr.size, arr.dtype, arr.ndim))
test_array1 = np.full((3,3),[4,2,3])
print("Test array1:: set shape")
printinfo(test_array1)
test_array2 = np.ones_like(test_array1)
print("Test array2:: ones_like(test_array1)")
printinfo(test_array2)
Test array1:: set shape
[[4 2 3]
[4 2 3]
[4 2 3]]
shape:: (3, 3), size::9 dtype::int64 dimension::2
Test array2:: ones_like(test_array1)
[[1 1 1]
[1 1 1]
[1 1 1]]
shape:: (3, 3), size::9 dtype::int64 dimension::2
ndarray를 만드는 대표적 함수를 정리해보았다. 다만, ndarray를 생성하는 것에는 위의 방법들만 한정되어있는 것이 아니다. Numpy API Refernce를 참고하면 아래와 같이 ndarray 생성에 활용되는 많은 함수가 존재한다.

'공부한 것들.. > Numpy' 카테고리의 다른 글
Numpy 집계 함수 (0) | 2024.09.12 |
---|---|
Numpy 기본 연산 (0) | 2024.09.12 |
Numpy ndarray shape 변경 (0) | 2024.09.12 |
Numpy :: 데이터 타입 (0) | 2024.09.12 |
Numpy 자료구조 :: ndarray (N- Dimension Array) (0) | 2024.09.09 |